Burning / Destroying Tokens and Collections
Put a temporary or permanent stop to token transfers.
"Burning" refers to the process of decreasing a token's supply and removing it from circulation, or in some cases, even removing the token from the blockchain entirely.
What you'll need:
- Some Enjin Coin to pay for Transaction Fees.
You can obtain cENJ (Canary ENJ) for testing from the Canary faucet.- An Enjin Platform Account.
- A Token to burn.
There are two ways to Burn a token:
Option A. Using the Enjin Dashboard
Burning token's supply
In the Platform menu, navigate to "Tokens".
Locate the token you wish to burn, click the 3 vertical dots (⋮) to it's right, then click the "Burn" button.
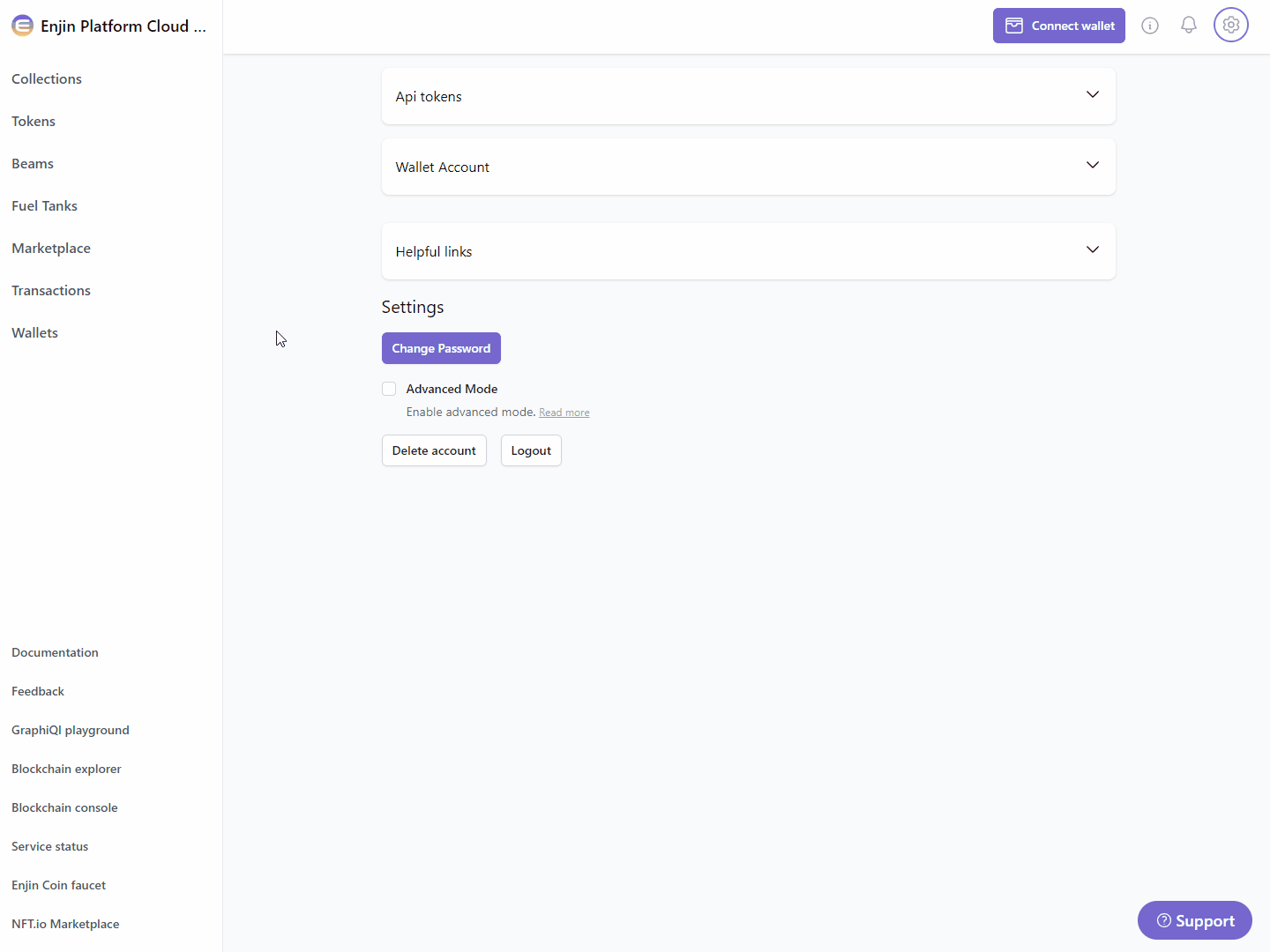
Insert the amount of tokens to Burn, and click on the "Burn" button.
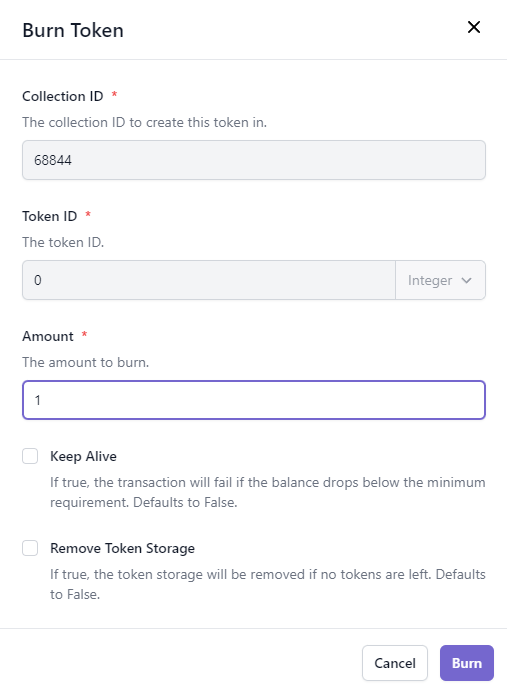
Burn form on Enjin Platform
The Transaction Request will then appear in the "Transactions" menu.

A notification appears after you create a Mutation.

Clicking "View" on the notification will take you to your Transactions List.
Since this transaction is a Mutation, you will need to sign the transaction using your Wallet.
- If a Wallet Daemon is running and configured, the transaction request will be signed automatically.
- If a wallet is connected such as the Enjin Wallet or Polkadot.js, the transaction must be signed manually by clicking the "Sign" button and approving the signature request in your wallet.
Destroying a token and removing it from the Blockchain
To destroy a token, these requirements must be met:
- The caller is the collection owner
- The token has no attributes
- If the token has attributes, you can remove the attributes by clicking the 3 vertical dots (⋮) next to the token, followed by "Attributes" and selecting "Remove All".
- The token has 0 supply
- If the token has supply, you can follow the above guide Burning token's supply to remove all token supply (as long as you own all of the token's supply)
Note - you can remove the supply and destroy the token in the same Burn transaction.
Burning a token and destroying it are two different actions.
The action demonstrated above is the action of burning a token, which decreases it's circulating supply.
While destroying a token removes the token from the blockchain, and retrieves the Storage Deposit to the collection owner.
To destroy a token, follow the above instructions for Burning a token, but make sure to tick the Remove Token Storage
box.

Destroying a collection
To destroy a collection, these requirements must be met:
- The caller is the collection owner
- The collection has no attributes
- If the collection has attributes, you can remove the attributes by clicking the 3 vertical dots (⋮) next to the collection, followed by "Attributes" and selecting "Remove All".
- The collection has 0 tokens in storage
- If the collection has some tokens, you can follow the above guide Destroying a token and removing it from the Blockchain for each of the tokens in the collection, to destroy them all.
In the Platform menu, navigate to "Collections".
Locate the collection you wish to destroy, click the 3 vertical dots (⋮) to it's right, then click the "Destroy" button.
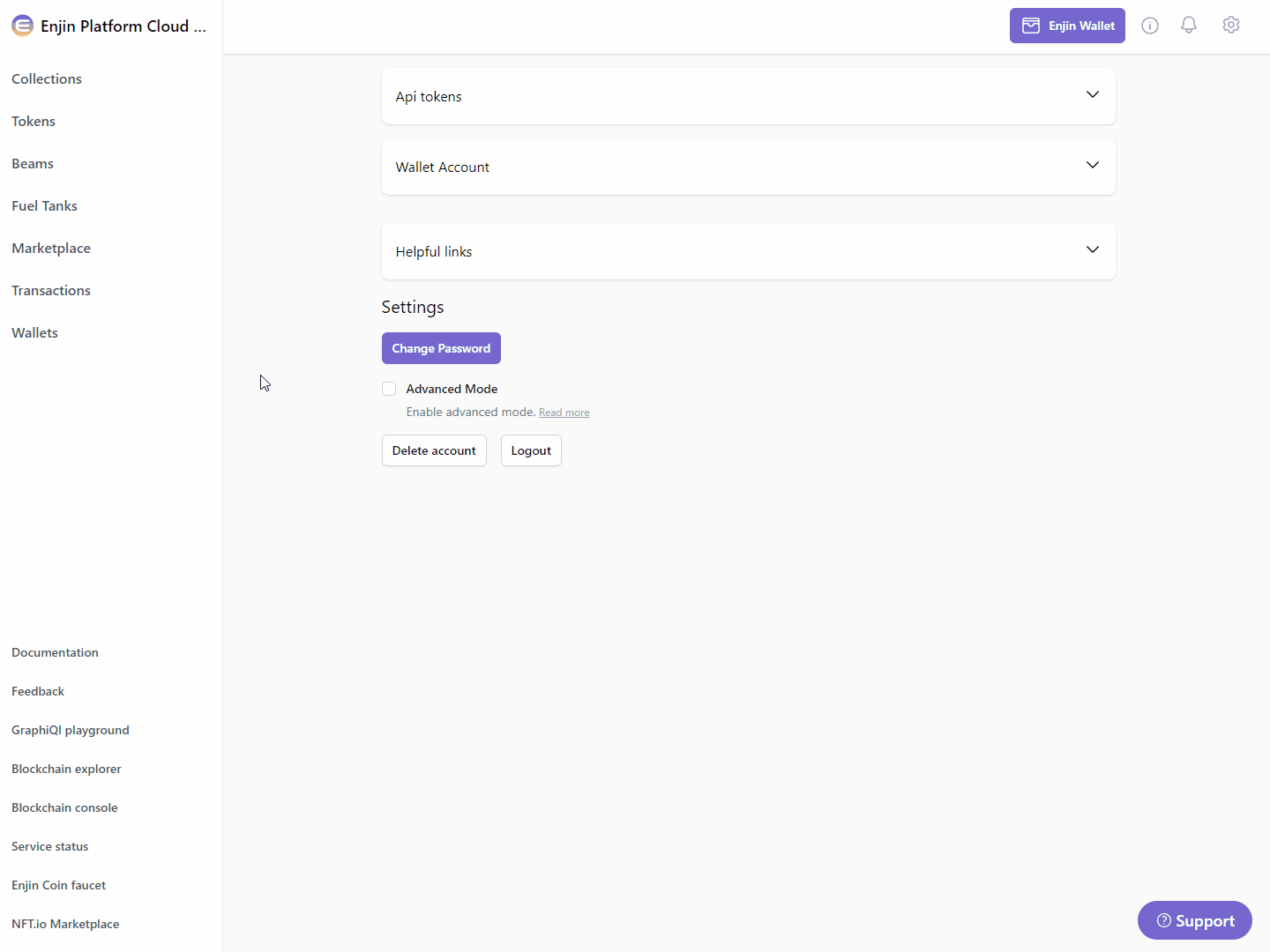
Then, confirm by clicking the "Destroy" button
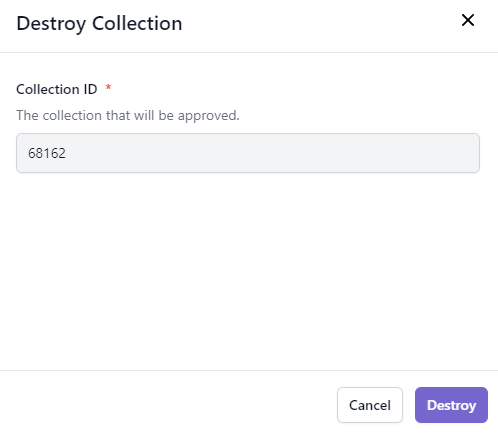
Destroy Collection form on Enjin Platform
The Transaction Request will then appear in the "Transactions" menu

A notification appears after you create a Mutation.

Clicking "View" on the notification will take you to your Transactions List.
Since this transaction is a Mutation, you will need to sign the transaction using your Wallet.
- If a Wallet Daemon is running and configured, the transaction request will be signed automatically.
- If a wallet is connected such as the Enjin Wallet or Polkadot.js, the transaction must be signed manually by clicking the "Sign" button and approving the signature request in your wallet.
Option B. Using the Enjin API & SDKs
Burning token's supply
Use the Burn
mutation:
mutation BurnToken{
Burn(
collectionId: 68844 #Specify the Collection ID
params: {
tokenId: {integer: 0} #Specify the Token ID
amount: 1 #Specify the amount of supply to burn
}
) {
id
method
state
}
}
Snippet In Progress
Snippet In Progress
fetch('https://platform.canary.enjin.io/graphql', {
method: 'POST',
headers: {'Content-Type': 'application/json','Authorization': 'Your_Platform_Token_Here'},
body: JSON.stringify({
query: `
mutation BurnToken(
$collection_id: BigInt!
$token_id: BigInt!
$amount: BigInt!
){
Burn(
collectionId: $collection_id
params: {
tokenId: {integer: $token_id}
amount: $amount
}
) {
id
method
state
}
}
`,
variables: {
collection_id: 36105, //Specify the collection ID
token_id: 5, //Specify the amount of supply to burn
amount: 1 //Specify the amount of supply to burn
}
}),
})
.then(response => response.json())
.then(data => console.log(data));
const axios = require('axios');
axios.post('https://platform.canary.enjin.io/graphql', {
query: `
mutation BurnToken(
$collection_id: BigInt!
$token_id: BigInt!
$amount: BigInt!
){
Burn(
collectionId: $collection_id
params: {
tokenId: {integer: $token_id}
amount: $amount
}
) {
id
method
state
}
}
`,
variables: {
collection_id: 36105, //Specify the collection ID
token_id: 5, //Specify the amount of supply to burn
amount: 1 //Specify the amount of supply to burn
}
}, {
headers: {'Content-Type': 'application/json','Authorization': 'Your_Platform_Token_Here'}
})
.then(response => console.log(response.data))
.catch(error => console.error(error));
import requests
query = '''
mutation BurnToken(
$collection_id: BigInt!
$token_id: BigInt!
$amount: BigInt!
){
Burn(
collectionId: $collection_id
params: {
tokenId: {integer: $token_id}
amount: $amount
}
) {
id
method
state
}
}
'''
variables = {
'collection_id': 36105, #Specify the collection ID
'token_id': 5, #Specify the amount of supply to burn
'amount': 1 #Specify the amount of supply to burn
}
response = requests.post('https://platform.canary.enjin.io/graphql',
json={'query': query, 'variables': variables},
headers={'Content-Type': 'application/json', 'Authorization': 'Your_Platform_Token_Here'}
)
print(response.json())
Once the transaction is executed, the token supply will be burned
Destroying a token and removing it from the Blockchain
To destroy a token, these requirements must be met:
- The caller is the collection owner
- The token has no attributes
- If the token has attributes, they can be removed using the
RemoveAllAttributes
mutation- The token has 0 supply
- You can remove the supply and destroy the token in the same Burn transaction.
Use the Burn
mutation, and add removeTokenStorage: true
property
mutation DestroyToken{
Burn(
collectionId: 68844 #Specify the Collection ID
params: {
tokenId: {integer: 0} #Specify the Token ID
amount: 1 #Specify the amount of supply to burn
removeTokenStorage: true
}
) {
id
method
state
}
}
Snippet In Progress
Snippet In Progress
fetch('https://platform.canary.enjin.io/graphql', {
method: 'POST',
headers: {'Content-Type': 'application/json','Authorization': 'Your_Platform_Token_Here'},
body: JSON.stringify({
query: `
mutation BurnToken(
$collection_id: BigInt!
$token_id: BigInt!
$amount: BigInt!
){
Burn(
collectionId: $collection_id
params: {
tokenId: {integer: $token_id}
amount: $amount
removeTokenStorage: true
}
) {
id
method
state
}
}
`,
variables: {
collection_id: 36105, //Specify the collection ID
token_id: 5, //Specify the amount of supply to burn
amount: 1 //Specify the amount of supply to burn
}
}),
})
.then(response => response.json())
.then(data => console.log(data));
const axios = require('axios');
axios.post('https://platform.canary.enjin.io/graphql', {
query: `
mutation BurnToken(
$collection_id: BigInt!
$token_id: BigInt!
$amount: BigInt!
){
Burn(
collectionId: $collection_id
params: {
tokenId: {integer: $token_id}
amount: $amount
removeTokenStorage: true
}
) {
id
method
state
}
}
`,
variables: {
collection_id: 36105, //Specify the collection ID
token_id: 5, //Specify the amount of supply to burn
amount: 1 //Specify the amount of supply to burn
}
}, {
headers: {'Content-Type': 'application/json','Authorization': 'Your_Platform_Token_Here'}
})
.then(response => console.log(response.data))
.catch(error => console.error(error));
import requests
query = '''
mutation BurnToken(
$collection_id: BigInt!
$token_id: BigInt!
$amount: BigInt!
){
Burn(
collectionId: $collection_id
params: {
tokenId: {integer: $token_id}
amount: $amount
removeTokenStorage: true
}
) {
id
method
state
}
}
'''
variables = {
'collection_id': 36105, #Specify the collection ID
'token_id': 5, #Specify the amount of supply to burn
'amount': 1 #Specify the amount of supply to burn
}
response = requests.post('https://platform.canary.enjin.io/graphql',
json={'query': query, 'variables': variables},
headers={'Content-Type': 'application/json', 'Authorization': 'Your_Platform_Token_Here'}
)
print(response.json())
Once the transaction is executed, the token will be destroyed and the Storage Deposit will be retrieved.
Destroying a collection
To destroy a collection, these requirements must be met:
- The caller is the collection owner
- The collection has no attributes
- If the collection has attributes, they can be removed using the
RemoveAllAttributes
mutation- The collection has 0 tokens in storage
- If the collection has some tokens, you can the above instructions for Destroying a token for each of the tokens in the collection, to destroy them all.
mutation DestroyCollection {
DestroyCollection(
collectionId: 68844 #Specify the Collection ID
) {
id
method
state
}
}
Snippet In Progress
Snippet In Progress
fetch('https://platform.canary.enjin.io/graphql', {
method: 'POST',
headers: {'Content-Type': 'application/json','Authorization': 'Your_Platform_Token_Here'},
body: JSON.stringify({
query: `
mutation DestroyCollection($collection_id: BigInt!){
DestroyCollection(
collectionId: $collection_id
) {
id
method
state
}
}
`,
variables: {
collection_id: 36105 //Specify the collection ID
}
}),
})
.then(response => response.json())
.then(data => console.log(data));
const axios = require('axios');
axios.post('https://platform.canary.enjin.io/graphql', {
query: `
mutation DestroyCollection($collection_id: BigInt!){
DestroyCollection(
collectionId: $collection_id
) {
id
method
state
}
}
`,
variables: {
collection_id: 36105 //Specify the collection ID
}
}, {
headers: {'Content-Type': 'application/json','Authorization': 'Your_Platform_Token_Here'}
})
.then(response => console.log(response.data))
.catch(error => console.error(error));
import requests
query = '''
mutation DestroyCollection($collection_id: BigInt!){
DestroyCollection(
collectionId: $collection_id
) {
id
method
state
}
}
'''
variables = {
'collection_id': 36105 #Specify the collection ID
}
response = requests.post('https://platform.canary.enjin.io/graphql',
json={'query': query, 'variables': variables},
headers={'Content-Type': 'application/json', 'Authorization': 'Your_Platform_Token_Here'}
)
print(response.json())
Once the transaction is executed, the collection will be destroyed.
Need to send a transaction request to user's wallet?
This can be done using Enjin Platform API & WalletConnect!
To learn more, check out the Using WalletConnect page.
Updated 11 days ago