Minting Tokens
Start minting your token's supply. You can mint it straight into your players' wallets.
Now that you've got your tokens created, it's time to start minting them into player wallets as they are earned.
We call this "Play-to-Mint," giving players the power to create tokens themselves, which feels more rewarding.
Plus, it keeps your processes more efficient by delivering tokens right to players' wallets without any unnecessary transfer
transactions.
What you'll need
- Some Enjin Coin on Enjin Matrixchain to pay for Transaction Fees and at least 0.01 ENJ for Storage Deposits.
You can obtain cENJ (Canary ENJ) for testing from the Canary faucet.- An Enjin Platform Account.
- A Collection and a Token to transfer.
There are two ways to use the Create Asset functionalities:
Option A. Using the Enjin Dashboard
In the Platform menu, navigate to "Tokens".
Locate the token you wish to mint, click the 3 vertical dots (⋮) to it's right, then click the "Mint" button.
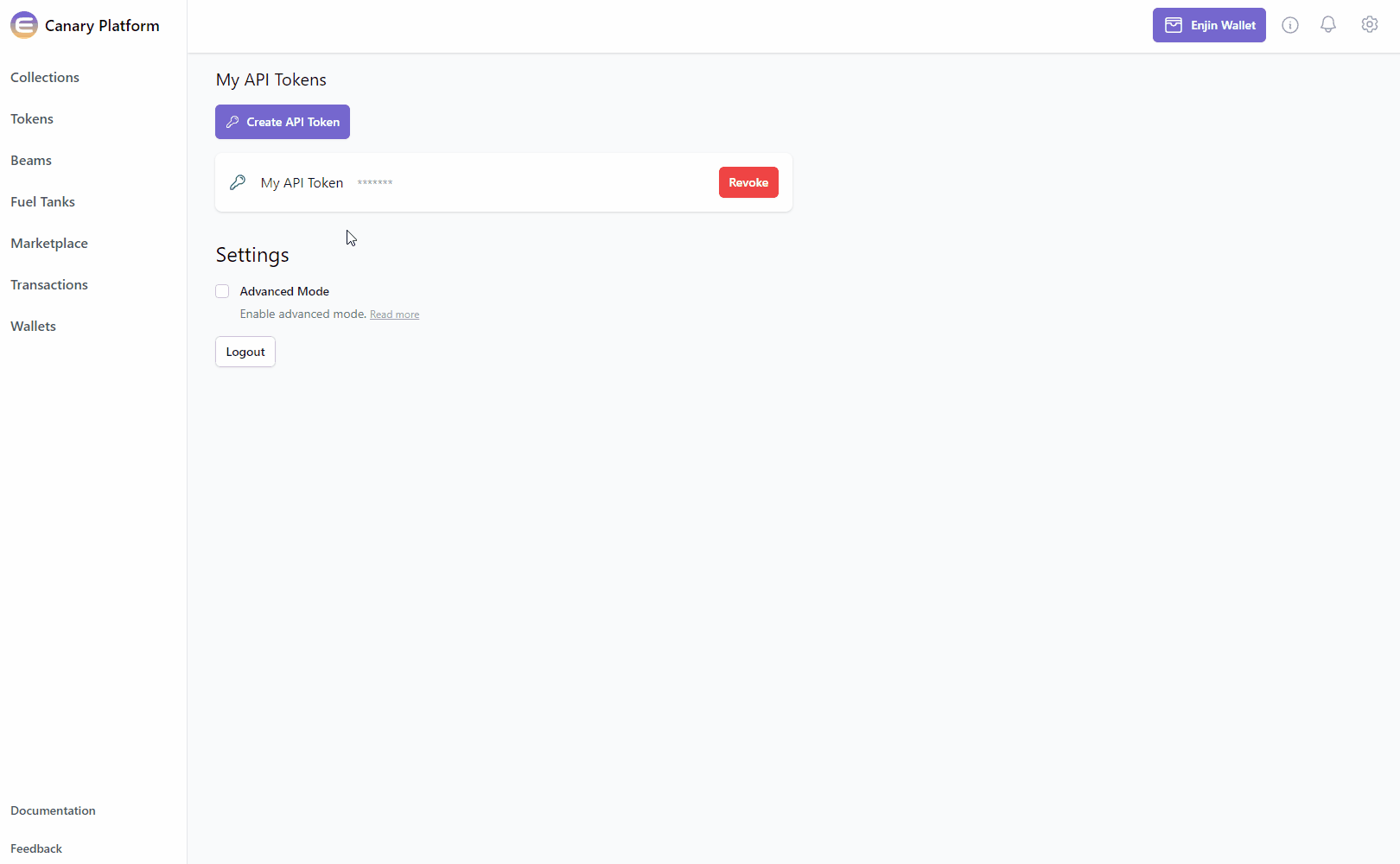
Set the recipient, amount and Unit Price in the corresponding fields, and Click on "Mint"
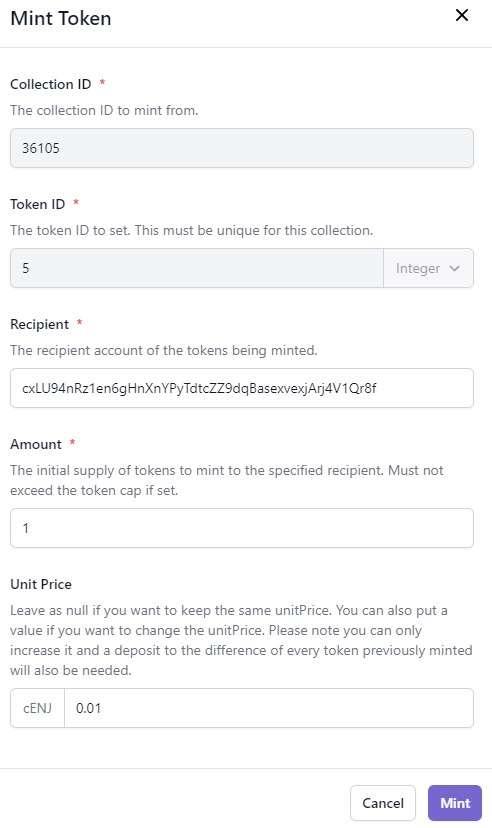
The Transaction Request will then appear in the "Transactions" menu.

A notification appears after you create a Mutation.

Clicking "View" on the notification will take you to your Transactions List.
Since this transaction is a Mutation, you will need to sign the transaction using your Wallet.
- If a Wallet Daemon is running and configured, the transaction request will be signed automatically.
- If a wallet is connected such as the Enjin Wallet or Polkadot.js, the transaction must be signed manually by clicking the "Sign" button and approving the signature request in your wallet.
Option B. Using the Enjin API & SDKs
The BatchMint mutation enables you to efficiently create multiple tokens within a single blockchain transaction. This process, known as batch minting, simplifies the minting of multiple tokens, reducing transaction fees and processing time.
mutation BatchMint {
BatchMint(
collectionId: "7154" #Specify the collection ID
recipients: [
{
account: "0xaa89f9099742a928051c41eadba188ad4e863539ff96f16722ae7850271c2921" #The recipient of the mint
mintParams: {
amount:1 #Amount to mint
tokenId: {integer: 6533} #Token ID to mint
}
}
]
) {
id
method
state
}
}
using System.Text.Json;
using Enjin.Platform.Sdk;
// Define the list of recipients and their mint parameters
var recipients = new List<MintRecipient>
{
new MintRecipient()
.SetAccount("0xaa89f9099742a928051c41eadba188ad4e863539ff96f16722ae7850271c2921")
.SetMintParams(new MintTokenParams()
.SetAmount(1)
.SetTokenId(new EncodableTokenIdInput().SetInteger(6533))
)
};
// Setup the mutation
var batchMint = new BatchMint()
.SetCollectionId(7154)
.SetRecipients(recipients.ToArray());
// Define and assign the return data fragment to the mutation
var batchMintFragment = new TransactionFragment()
.WithId()
.WithMethod()
.WithState();
batchMint.Fragment(batchMintFragment);
// Create and auth a client to send the request to the platform
var client = PlatformClient.Builder()
.SetBaseAddress("https://platform.canary.enjin.io")
.Build();
client.Auth("Your_Platform_Token_Here");
// Send the request and write the output to the console.
// Only the fields that were requested in the fragment will be filled in,
// other fields which weren't requested in the fragment will be set to null.
var response = await client.SendBatchMint(batchMint);
Console.WriteLine(JsonSerializer.Serialize(response.Result.Data));
#include "EnjinPlatformSdk/CoreMutations.hpp"
#include <iostream>
using namespace enjin::platform::sdk;
using namespace std;
int main() {
// Define the list of recipients and their mint parameters
shared_ptr tokenId = make_shared<EncodableTokenIdInput>();
tokenId->SetInteger(make_shared<SerializableString>("0"));
MintTokenParams mintTokenParams = MintTokenParams()
.SetAmount(make_shared<SerializableString>("1"))
.SetTokenId(tokenId);
MintRecipient mintRecipient = MintRecipient()
.SetAccount(make_shared<SerializableString>("0xaa89f9099742a928051c41eadba188ad4e863539ff96f16722ae7850271c2921"))
.SetMintParams(make_shared<MintTokenParams>(mintTokenParams));
vector<MintRecipient> recipients;
recipients.push_back(mintRecipient);
// Setup mutation
BatchMint batchMint = BatchMint()
.SetCollectionId(make_shared<SerializableString>("7154"))
.SetRecipients(make_shared<SerializableArray<MintRecipient>>(recipients));
// Define and assign the return data fragment to the mutation
shared_ptr<TransactionFragment> transactionFragment = make_shared<TransactionFragment>();
transactionFragment
->WithId()
.WithMethod()
.WithState();
batchMint.SetFragment(transactionFragment);
// Create and auth a client to send the request to the platform
unique_ptr<PlatformClient> client = PlatformClient::Builder()
.SetBaseAddress("https://platform.canary.enjin.io")
.Build();
client->Auth("Your_Platform_Token_Here");
// Send the request then get the response and write the output to the console.
// Only the fields that were requested in the fragment will be filled in,
// other fields which weren't requested in the fragment will be set to null.
future<shared_ptr<IPlatformResponse<GraphQlResponse<Transaction>>>> futureResponse = SendBatchMint(*client, batchMint);
// Get the platform response holding the HTTP data
PlatformResponsePtr<GraphQlResponse<Transaction>> response = futureResponse.get();
// Get the result, a GraphQL response, holding the GraphQL data
const optional<GraphQlResponse<Transaction>>& gqlResult = response->GetResult();
// Write the result data to the console
if (gqlResult.has_value() && gqlResult->IsSuccess())
{
const optional<Transaction>& transaction = gqlResult->GetData()->GetResult();
std::cout << to_string(transaction->GetId().value()) << std::endl;
std::cout << ToString(transaction->GetMethod().value()) << std::endl;
}
// Write any error messages to the console
if (gqlResult.has_value() && gqlResult->HasErrors())
{
const optional<vector<GraphQlError>>& errors = gqlResult->GetErrors();
for (const GraphQlError& error : errors.value()) {
std::cout << error.GetMessage().value() << std::endl;
}
}
client.reset();
return 0;
}
fetch('https://platform.canary.enjin.io/graphql', {
method: 'POST',
headers: {'Content-Type': 'application/json','Authorization': 'Your_Platform_Token_Here'},
body: JSON.stringify({
query: `
mutation BatchMint(
$collection_id: BigInt!
) {
BatchMint(
collectionId: $collection_id
recipients: [
{
account: "0xaa89f9099742a928051c41eadba188ad4e863539ff96f16722ae7850271c2921" #The recipient of the mint
mintParams: {
amount:1 #Amount to mint
tokenId: {integer: 6533} #Token ID to mint
}
}
]
) {
id
method
state
}
}
`,
variables: {
collection_id: 7154 //Specify the collection ID
}
}),
})
.then(response => response.json())
.then(data => console.log(data));
const axios = require('axios');
axios.post('https://platform.canary.enjin.io/graphql', {
query: `
mutation BatchMint(
$collection_id: BigInt!
) {
BatchMint(
collectionId: $collection_id
recipients: [
{
account: "0xaa89f9099742a928051c41eadba188ad4e863539ff96f16722ae7850271c2921" #The recipient of the mint
mintParams: {
amount:1 #Amount to mint
tokenId: {integer: 6533} #Token ID to mint
}
}
]
) {
id
method
state
}
}
`,
variables: {
collection_id: 7154 //Specify the collection ID
}
}, {
headers: {'Content-Type': 'application/json','Authorization': 'Your_Platform_Token_Here'}
})
.then(response => console.log(response.data))
.catch(error => console.error(error));
import requests
query = '''
mutation BatchMint(
$collection_id: BigInt!
) {
BatchMint(
collectionId: $collection_id
recipients: [
{
account: "0xaa89f9099742a928051c41eadba188ad4e863539ff96f16722ae7850271c2921" #The recipient of the mint
mintParams: {
amount:1 #Amount to mint
tokenId: {integer: 6533} #Token ID to mint
}
}
]
) {
id
method
state
}
}
'''
variables = {
'collection_id': 7154 #Specify the collection ID
}
response = requests.post('https://platform.canary.enjin.io/graphql',
json={'query': query, 'variables': variables},
headers={'Content-Type': 'application/json', 'Authorization': 'Your_Platform_Token_Here'}
)
print(response.json())
A WebSocket event will also be fired so you can pick up the transfer transaction in real time by listening to the app channel on the WebSocket.
More Fields and Arguments Available!
While the examples here cover the core functionalities of the
BatchMint
mutation, there are a few more settings you can adjust.To view and understand all the available settings for the
BatchMint
mutation, refer to our GraphQL Schema on Apollo.This resource will guide you in tailoring the
BatchMint
mutation to your specific requirements.For instance, instead of batch minting with the MintParams object, you can batch create new tokens with the CreateParams object.
You've minted a token!
What if you need to transfer a token? proceed to Transferring Tokens.
Updated about 13 hours ago